The PowerShell Confirm parameter is an essential safeguard that prompts users before performing potentially destructive actions. This article will explore how to implement the -Confirm
parameter in custom PowerShell functions to make them more robust and user-friendly.
What is the PowerShell Confirm parameter?
The -Confirm
parameter safeguards against actions that change a system, environment, or data. It works by prompting the user for confirmation before PowerShell executes the command. Including this parameter and functionality in your functions provides users with an additional layer of safety when running your script and functions.

Adding the PowerShell Confirm parameter
The -Confirm
parameter is made possible in advanced functions through the CmdletBinding
attribute. The CmdletBinding
attribute provides compiled cmdlet-like features to your functions.
Read More: PowerShell Advanced Functions: Getting Started Guide | Jeff Brown Tech
Read More: Using PowerShell WhatIf So You Don’t Break Stuff | Jeff Brown Tech
Here is an example function named Remove-EmployeeData
with the CmdletBinding
attribute, including the SupportsShouldProcess
property.
The CmdletBinding
attribute also enables access to the $PSCmdlet
automatic variable. You call the $PSCmdlet.SupportShouldProcess()
method for PowerShell to check for the -Confirm
parameter before processing the function logic.
function Remove-EmployeeData {
[CmdletBinding(SupportsShouldProcess)]
param (
[Parameter(Mandatory)]
[string]$Name
)
if ($PSCmdlet.ShouldProcess($Name)) {
"Removing data for $Name..."
# Logic to remove the data
}
}
If you include the -Confirm parameter, PowerShell prompts for confirmation before making the change.
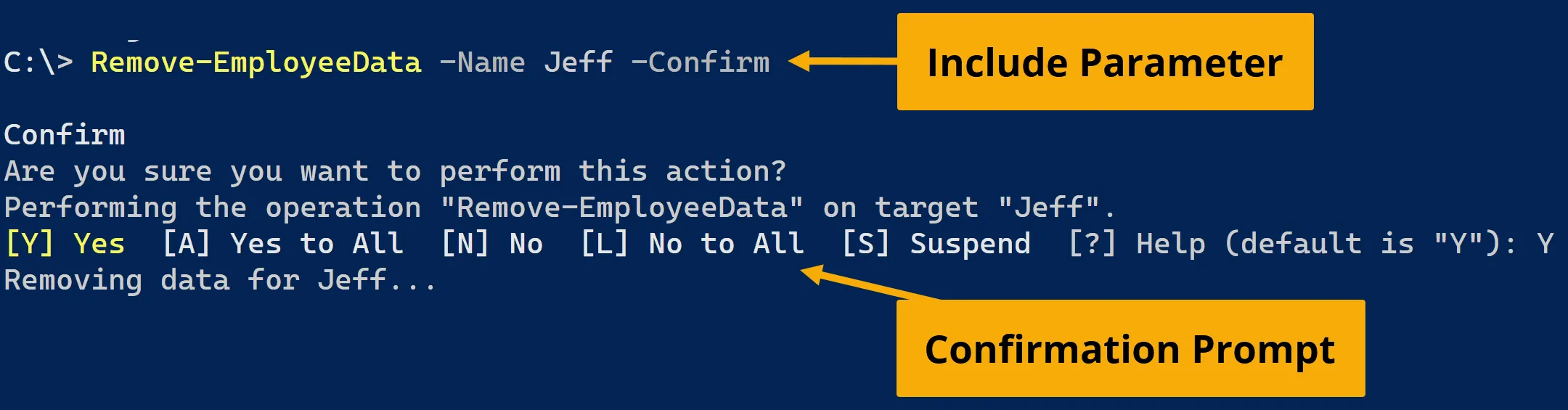
Setting ConfirmImpact
The above example relies on the user adding the -Confirm
parameter to invoke the confirmation prompt. However, you can display confirmation prompts without specifying the parameter by setting the ConfirmImpact
property to “High” in CmdletBinding
. Adding this property prompts the user as if using the -Confirm
parameter.
Review the updated CmdletBinding
attribute below.
function Remove-EmployeeData {
[CmdletBinding(
SupportsShouldProcess,
ConfirmImpact = "High"
)]
# ...
Calling the function now without -Confirm
still prompts for confirmation because of the High
impact.

However, you can bypass the prompt by adding -Confirm:$false
to the function execution, like this:

$ConfirmPreference automatic variable
$ConfirmPreference
is a PowerShell automatic variable that determines when ConfirmImpact
displays confirmation prompts. The possible values for $ConfirmPreference
and ConfirmImpact
include:
- High
- Medium
- Low
- None
By default, $ConfirmPreference
is set to High
while ConfirmImpact
is set to Medium
.
If ConfirmImpact
is set equal to or higher than $ConfirmPreference
, then the function prompts the user for confirmation. Otherwise, PowerShell does not display a confirmation prompt. Here is a table with a few examples outlining this logic.
ConfirmImpact | $ConfirmPreference | Result |
---|---|---|
High | High | Display prompt |
Medium | High | No prompt |
Medium | Medium | Display prompt |
High | Medium | Display prompt |
Summary
The -Confirm
parameter is an invaluable tool when writing PowerShell functions, especially those that make changes to the system or environment. Incorporating this parameter into your scripts and functions makes them more versatile, robust, and user-friendly – key traits of any good PowerShell function.