Advanced functions take your PowerShell development to the next level, letting you create cmdlet-like scripts with advanced features. In this article, we’ll dive into what makes cmdlets and advanced functions essential in PowerShell, with examples and tips to help you master them.
What is a cmdlet?
A PowerShell cmdlet (pronounced “command-let”) performs a specific task in a PowerShell environment. Cmdlets are written in a .NET language, like C#, and compiled into a binary cmdlet. Cmdlets are the core building blocks of PowerShell and are often packaged into modules for easy installation and distribution.
Cmdlets have a few key features:
- Object-Oriented: Cmdlets operate on objects, not text. They can take input objects, process them, and return output objects, making working with structured data much more effortless.
- Verb-Noun Naming Convention: Cmdlets following a consistent naming convention of
Verb-Noun
. The naming convention provides clarity in what the cmdlet is doing, making them intuitive and easy to discover using tools likeGet-Command
. - Pipeline Integration: Cmdlets work in a pipeline, where the output of one cmdlet is passed to another cmdlet. Pipelines allow chaining commands together to perform complex tasks.
- Built-In Help: Cmdlets include documentation on how to use the cmdlet via
Get-Help
. - Lightweight and Task-Specific: Cmdlets are designed to be lightweight and perform specific tasks.
What are PowerShell advanced functions?
PowerShell advanced functions are script-based functions that behave like cmdlets and use advanced features such as common parameters, input processing, and access to additional methods. They allow PowerShell developers to write cmdlet-like functions without using a .NET language.
CmdletBinding Attribute
Advanced functions are made possible through the CmdletBinding
attribute. This attribute signals to PowerShell that the function should behave like a cmdlet. By adding [CmdletBinding()]
, the function can handle pipeline input, and you also gain access to common parameters like -Verbose
, -ErrorAction
, and more.
Here is an example function named New-Greeting
that includes the CmdletBinding
attribute and an empty param()
block.
function New-Greeting {
[CmdletBinding()]
param()
"Hello, World!"
}
The CmdletBinding
attribute includes several properties:
- ConfirmImpact: Specifies if the function action should be confirmed using the ShouldProcess method and displays a confirmation prompt before continuing.
- DefaultParameterSetName: Specifies the name of the default parameter set when PowerShell cannot determine which parameter set to use.
- HelpURI: Defines the online version of the function help topic.
- SupportsPaging: Adds the First, Skip, and IncludeTotalCount parameters to the function.
- SupportShouldProcess: Add the Confirm and WhatIf parameters to the function.
- PositionalBinding: Determines whether or not the function parameters are positional by default. The default value is
$True
.
Common Parameters
Common parameters are a set of cmdlet parameters you can use with any cmdlet. You do not need to implement them yourself as they are automatically implemented by PowerShell when including the CmdletBinding
attribute. Common parameters include:
- Debug
- ErrorAction
- ErrorVariable
- InformationAction
- InformationVariable
- OutVariable
- OutBuffer
- PipelineVariable
- ProgressAction
- Verbose
- WarningAction
- WarningVariable
While these parameters are available, they may not affect your advanced parameter. For example, if your function does not produce any verbose output, adding the Verbose
parameter will not affect it. For an example, see the Write methods section later in this article.
➡️ Read More: Common Parameter descriptions
Advanced Function Methods
Functions using the CmdletBinding
attribute can access additional methods and properties through the $PSCmdlet
variable. These methods include input processing, ShouldProcess
, ShouldContinue
, and ThrowTerminatingError
methods, and several Write
methods.
Input processing
Input processing methods include the begin
, process
, and ends
blocks. PowerShell 7.3 also adds a clean
block process method. You are not required to use any of these blocks in your function, and PowerShell puts the code in the end block if you do not use a named block. However, if you use any of the three blocks or define a dynamicparam
block, you must put all the function code in a named block.
➡️ Read More: PowerShell Begin Process End Blocks Demystified
Confirmation methods
With advanced functions, you can request user confirmation before the function performs an action that changes a system or resource. You can also add the WhatIf parameter that shows what changes the function will make.
➡️ Read More: Using PowerShell WhatIf So You Don’t Break Stuff
Error methods
You can use two different methods when errors occur. For non-terminating errors, you use the WriteError
method or the Write-Error
cmdlet. For terminating errors, you call the ThrowTerminatingError
method or use the throw
statement.
➡️ Read More: Mastering PowerShell Try Catch with Exception Messages
Write methods
Advanced functions can use different Write
methods to return different types of output. When using these methods, not all output goes to the next command in the pipeline. Various Write
methods include Write-Verbose
, Write-Warning
, Write-Error
, and Write-Progress
.
For example, here is a custom advanced function named New-Greeting
with one string parameter Name
with a default value of World. An if else
statement checks if the value of Name
is set to the default and uses Write-Verbose
to output a message.
function New-Greeting {
[CmdletBinding()]
param(
[Parameter()]
[string]
$Name = "World"
)
if ($Name -eq "World") {
Write-Verbose -Message "You are using the default Name value."
}
else {
Write-Verbose -Message "You are using a custom Name value."
}
"Hello, $Name!"
}
The screenshot below shows executing the function with and without specifying the Name
parameter and Write-Verbose
along with the changes in console output.
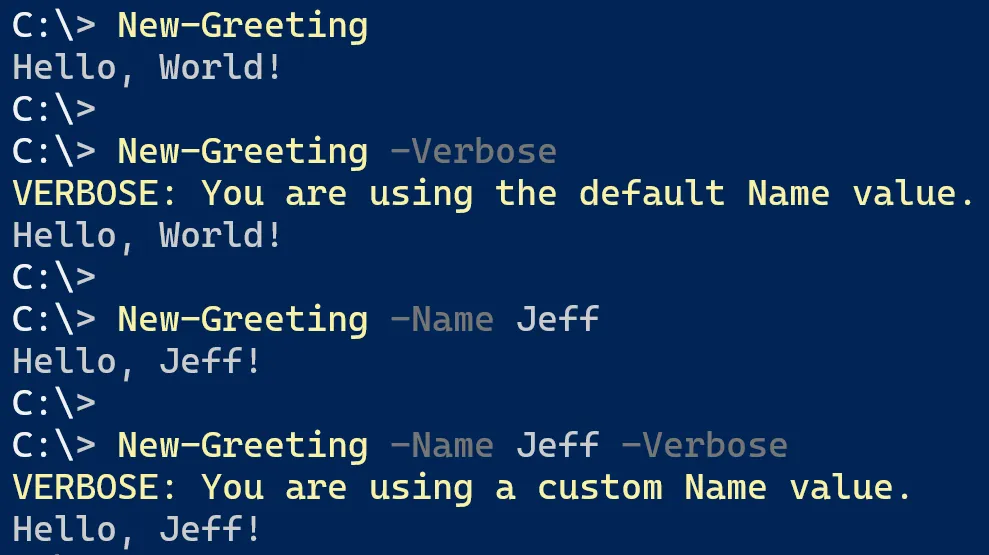
Here is another example of how to display custom messages using Write-Warning
inside a try catch
block for error handling.
try {
New-Item -Path C:\doesnotexist `
-Name myfile.txt `
-ItemType File `
-ErrorAction Stop
}
catch {
Write-Warning $Error[0]
}
PowerShell Advanced Functions Summary
Advanced functions in PowerShell provide developers and administrators with a powerful tool for building reusable, reliable, and professional-grade scripts. With components such as input processing, confirmation methods, error methods, and write methods, advanced functions enable you to build scripts that behave like cmdlets, allowing for robust automation and system management.
By mastering advanced functions, you can significantly enhance the functionality of your PowerShell scripts, taking full advantage of the language’s offerings.