You have had it happen before. You run a PowerShell script, and suddenly the console is full of errors. Did you know you can handle these errors in a much better way? Enter PowerShell try catch blocks!
Using error handling with PowerShell try catch blocks allows for managing and responding to these terminating errors. In this post, you will be introduced to PowerShell try catch blocks and learn how to handle specific exception messages.
Understanding PowerShell Try Catch Syntax
The PowerShell try catch block syntax is straightforward. It is composed of two sections enclosed in curly brackets. The first identified section is the try
block, and the second section is the catch
block.
try {
# Command(s) to try
}
catch {
# What to do with terminating errors
}
The try block can have as many statements in it as you want; however, keep the statements to as few as possible, probably just a single statement. The point of error handling is to work with one statement at a time and deal with anything that occurs from the error.
Here is an example of an error occurring in the PowerShell console. The command is creating a new file using the New-Item cmdlet and specifying a non-existent folder for Path.
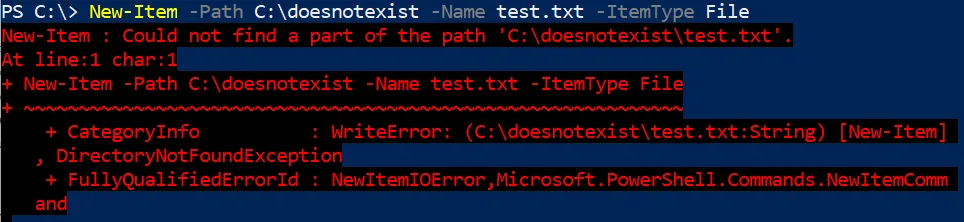
If this command was in a script, the output wastes some screen space, and the problem may not be immediately visible. Using a PowerShell try catch block, you can manipulate the error output and make it more readable.
Here is the same New-Item command in a try catch block. Note that line 5 uses the -ErrorAction parameter with a value of Stop to the command. Not all errors are considered “terminating,” so sometimes you need to add this bit of code to terminate into the catch block properly.
try {
New-Item -Path C:\doesnotexist `
-Name myfile.txt `
-ItemType File `
-ErrorAction Stop
}
catch {
Write-Warning -Message "Oops, ran into an issue"
}
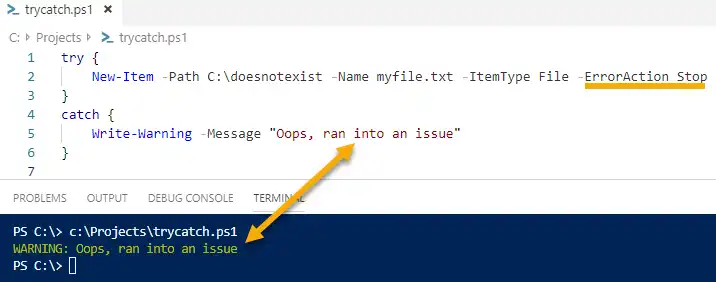
Instead of a block of red angry-looking text, you have a simple warning message that it ran into an issue. The non-existent Path name along with forcing -ErrorAction Stop drops the logic into the catch block and displays the custom warning.
Adding the $Error Variable to Catch Output
While more readable, this is not very helpful. All you know is the command did not complete successfully, but you don’t know why. Instead of displaying my custom message, you can display the specific error message that occurred instead of the entire exception block.
When an error occurs in the try block, it is saved to the automatic variable named $Error. The $Error variable contains an array of recent errors, and you can reference the most recent error in the array at index 0.
try {
New-Item -Path C:\doesnotexist `
-Name myfile.txt `
-ItemType File `
-ErrorAction Stop
}
catch {
Write-Warning $Error[0]
}
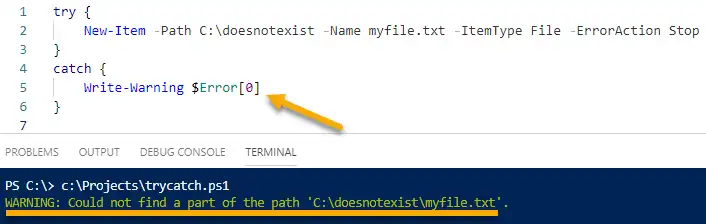
The warning output is now more descriptive showing that the command failed because it couldn’t find part of the path. This message was a part of our original error message but is now more concise.
Alternatively, you can save the incoming error to a variable using $_
. The dollar sign + underscore in PowerShell indicates the current item in the pipeline. In this case, the current item is the error message coming out of the try block.
Once you have saved this incoming message, you use it in a custom output message, like so:
try {
New-Item -Path C:\doesnotexist `
-Name myfile.txt `
-ItemType File `
-ErrorAction Stop
}
catch {
$message = $_
Write-Warning "Something happened! $message"
}
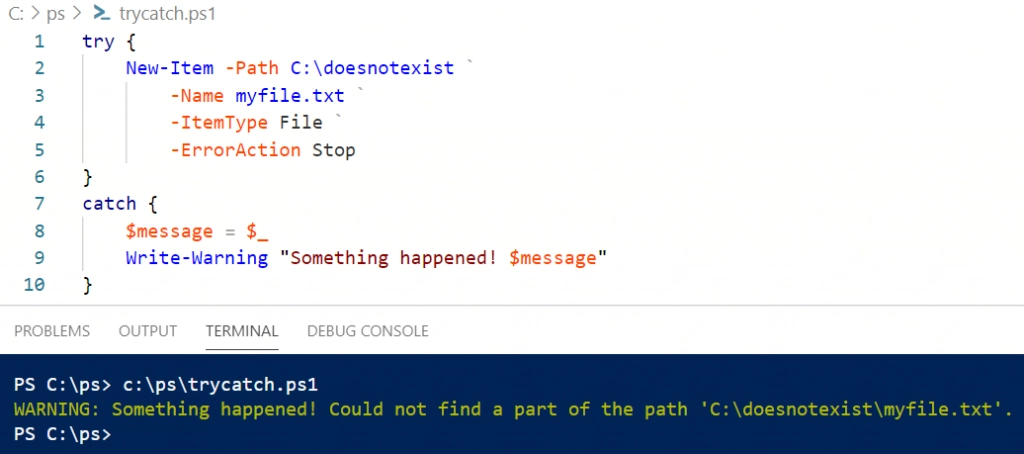
Adding Exception Messages to Catch Blocks
You can also use multiple catch blocks to handle specific errors differently. This example displays two different custom messages:
- One for if the path does not exist
- One for if an illegal character is used in the name
Note that the following screenshot shows the script running twice with two different commands in the try block. Each command, catch block, and the orange and green arrows indicate the final output.
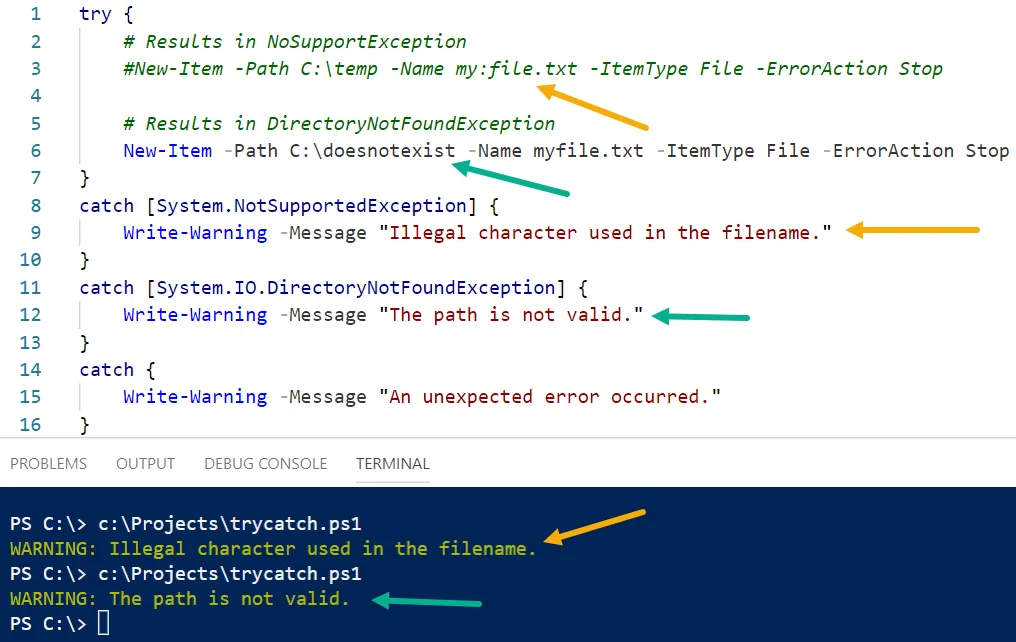
Looking at lines 14-16, there is a third catch block without an exception message. This is a “catch-all” block that will run if the error does not match any other exceptions. If you are running this script and seeing the message in the last catch block, you know the error is not related to an illegal character in the file name or part of the path not being valid.
Now how do you find the exception messages to use in the first two catch blocks? You find it by looking at the different information attached to the $Error
variable. After a failed command occurs, you can run $Error[0].Exception.GetType().FullName to view the exception message for the last error that occurred.
Going back to the PowerShell console, rerun the New-Item
command with a non-existent path, then run the $Error command to find the exception message.
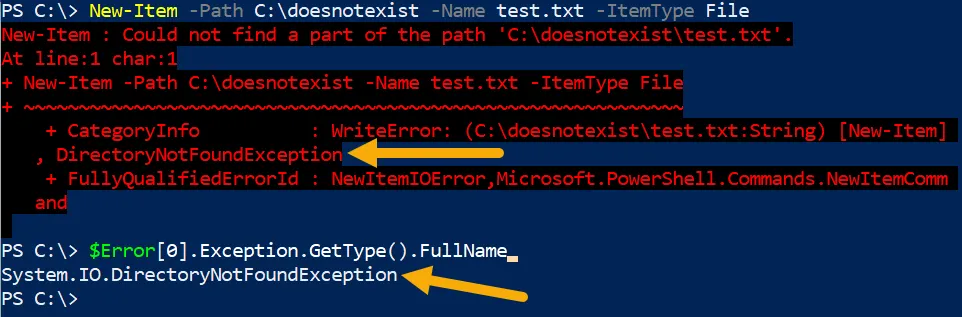
The red text immediately following the failed command also contains the exception message but does not contain which module it is from. Looking at the $Error variable shows the full message to be used for a catch block.
Getting Exception Messages in PowerShell 7
PowerShell version 7 introduced the Get-Error
command. This command displays the most recent error from the current session. After encountering an error, run Get-Error to show the exception type to use in the catch
block.
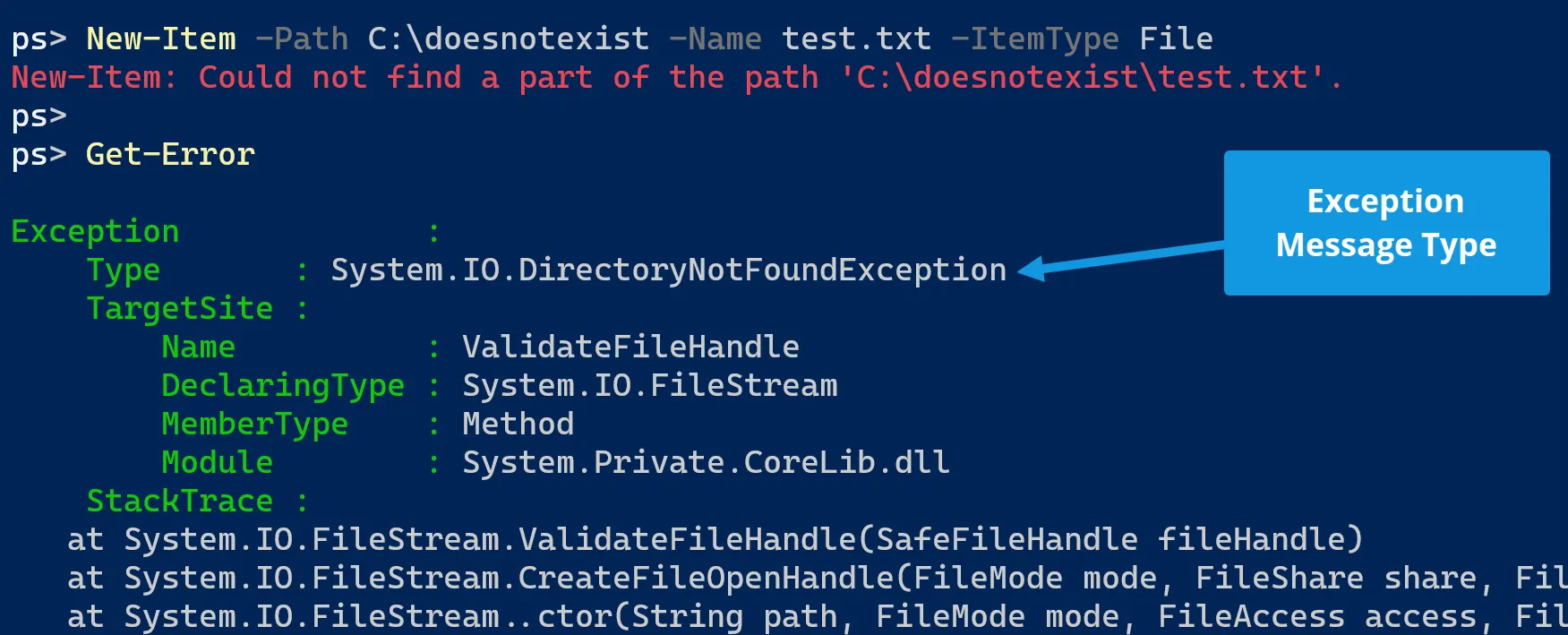
Summary
Using PowerShell try catch blocks gives additional power to handle errors in a script and take different actions based on the error. The catch block can display more than just an error message. It can contain logic that will resolve the error and continue executing the rest of the script.
Do you have any tips on using try/catch blocks? Leave a comment below or find me on Twitter or LinkedIn for additional discussion.
Enjoyed this article? Check out more of my PowerShell articles here!
References:
Microsoft Docs: About Try Catch Finally
Microsoft Docs: About Automatic Variables ($Error)
Microsoft Blog: Understanding Non-Terminating Errors in PowerShell