PowerShell script parameters are vital components that enable the customization and versatility of scripts, functions, and cmdlets. PowerShell parameters enhance the usability and adaptability of scripts by allowing users to pass information into the code instead of hardcoding values. Understanding how to define, manipulate, and leverage PowerShell parameters is essential for proficient scripting in PowerShell.
What are PowerShell Parameters?
PowerShell parameters provide input to scripts, functions, and cmdlets. Passing information into your code instead of hardcoding values makes the code more versatile and easier to use.
When using Get-Help to view a cmdlet’s documentation, you can filter down to view only parameter information using -Parameter
and a wildcard ( *
). Note some of the parameter properties, such as Required?
, Position?
, and Aliases
. You’ll learn more about these parameter attributes later in this tutorial.
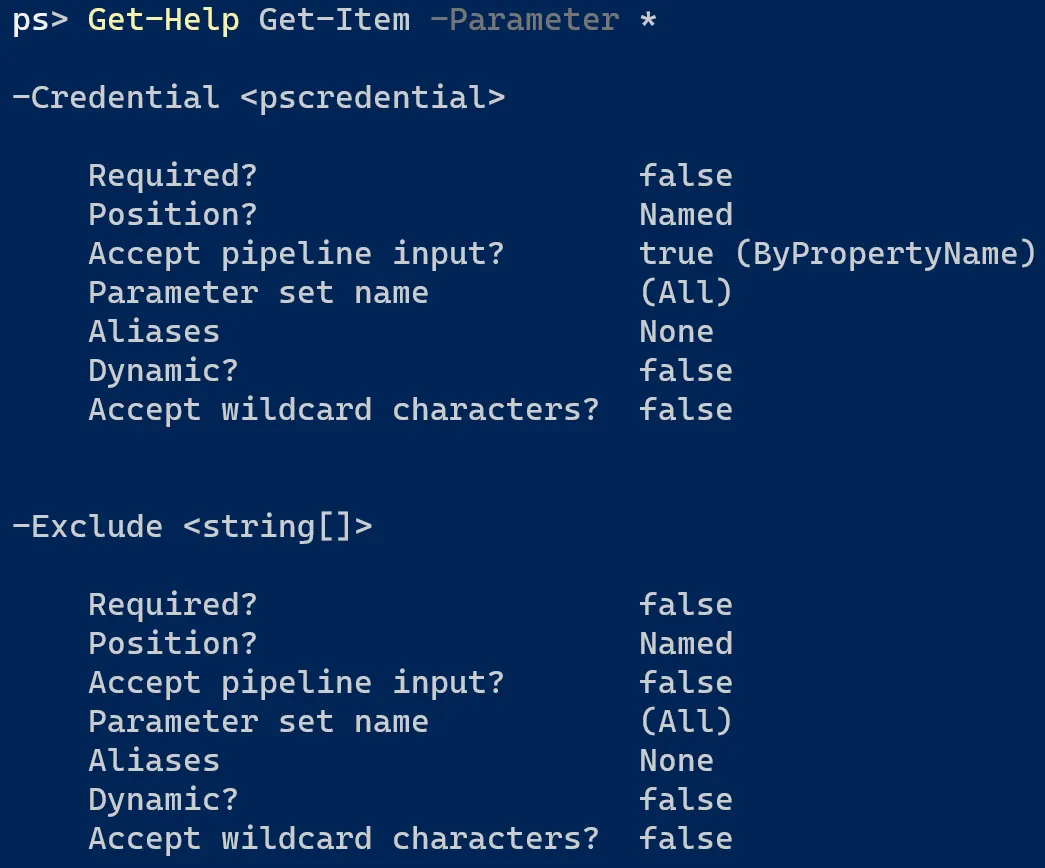
Defining Parameters
You define parameters using the param()
keyword along with a dollar sign ($
) and a parameter name, just like a variable. The example below is a parameter definition for a script name Add-TwoNumbers.ps1
along with statements showing the parameter values. Reference the parameters in your script just like a variable.
param(
$FirstNumber,
$SecondNumber
)
"$FirstNumber plus $SecondNumber equals $($FirstNumber + $SecondNumber)."
The screenshot below demonstrates calling the Add-TwoNumbers.ps1
script, along with the -FirstNumber
and -SecondNumber
parameters, passing the values 5
and 10
respectively.

Fantastic! However, in the current state, the parameters will accept any data type, such as strings. Here’s an example of passing strings as parameter values the result:

To avoid this, you make each parameter a specific data type (this is known as strongly-typed). Assigning data types to parameters ensures the user passes the expected data type for the script to be successful.
To add a data type, add square brackets ([ ]
) in front of the parameter name along with the data type. In the updated script code below, the parameters are double
to accept whole (integer) and decimal numbers.
Reference: PowerShell Data Types
param(
[double]$FirstNumber,
[double]$SecondNumber
)
"$FirstNumber plus $SecondNumber equals $($FirstNumber + $SecondNumber)."
The screenshot below shows script execution with integers, decimals, and a string. The last example fails as five
is not valid for the double data type, leading the script to fail.
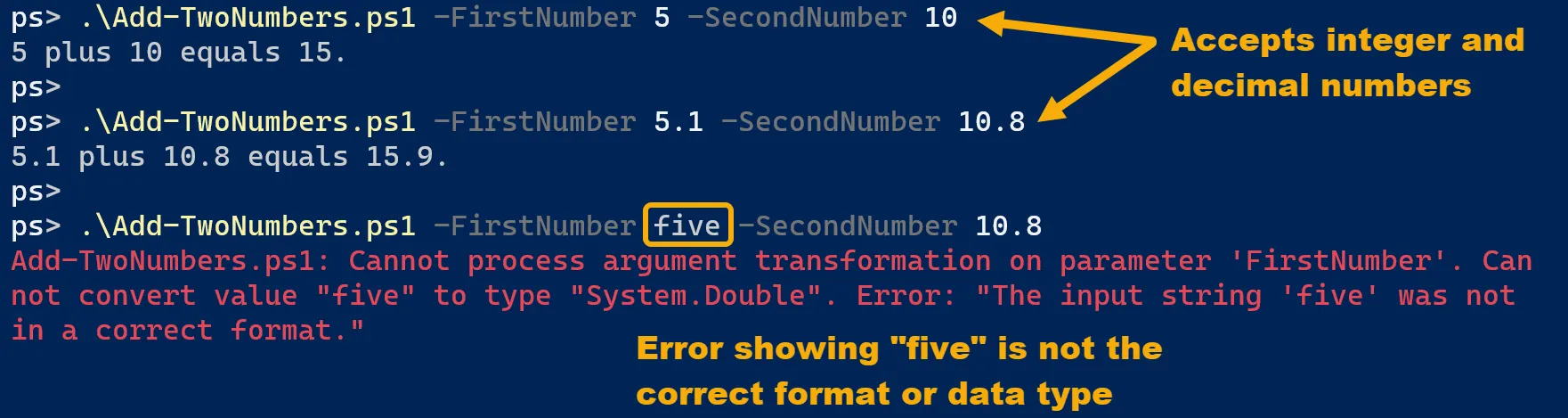
Parameter Attributes
You can define additional properties on a parameter through parameter attribute declaration. Add the statement [Parameter()]
before the parameter data type and name like this:
param(
[Parameter()]
[double]$FirstNumber,
[Parameter()]
[double]$SecondNumber
)
While there are many attributes you can define for a parameter, this tutorial focuses on two: mandatory and position.
Mandatory
Making a mandatory parameter means you must specify a parameter value when executing the script. Without the mandatory designation, the parameter is optional. If you run the Add-TwoNumbers.ps1 script without one or both parameters, the script will still run but may not give the expected results. The code depends on the user executing the script and providing both values.
Add the keyword Mandatory
to the [Parameter()]
attribute for both parameters in the script to make them mandatory.
Note: Older versions of PowerShell required the syntax Mandatory=$true
in the parameter attribute. However, this was simplified to just Mandatory
in later versions.
param(
[Parameter(Mandatory)]
[double]$FirstNumber,
[Parameter(Mandatory)]
[double]$SecondNumber
)
If you attempt to run the script without specifying these parameter values now, PowerShell will prompt you for the values.
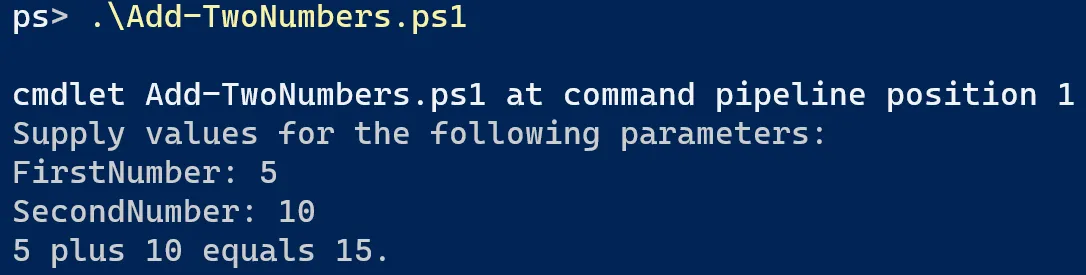
Position
The position parameter property specifies the order in which PowerShell evaluates parameters when the parameter is not explicitly specified. An example of this is the Rename-Item cmdlet. Below are two examples of using this cmdlet. The first uses the parameter names, and the second does not.
However, the -Path parameter has a position value of 0, and the -NewName parameter has a position value of 1. This designation means that the first parameter value is automatically assigned to -Path, and the second parameter value is assigned to -NewName.
# Using explicit parameter names
Rename-Item -Path myFile.txt -NewName yourFile.txt
# Using parameter positions
Rename-Item myFile.txt yourFile.txt
PowerShell automatically assigns positions based on the order in which you define the parameters in the param() block. As the script currently stands, you can assign each parameter value using position like this:
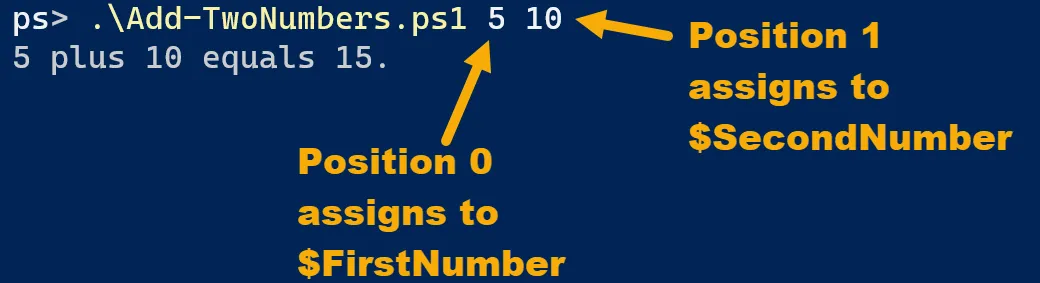
However, you can override this with a specific position using the Position
keyword in the parameter attribute section. The example code below puts $SecondNumber in Position 0 and $FirstNumber in Position 1.
Also, note the formatting change in the parameter attribute section. As you add more parameter attributes, place each one on a new line separated by commas.
param(
[Parameter(
Mandatory,
Position = 1
)]
[double]$FirstNumber,
[Parameter(
Mandatory,
Position = 0
)]
[double]$SecondNumber
)
Running the script now without parameters assigns 5
to $SecondNumber
while 10
becomes $FirstNumber
. Note the reversal in the output string from the previous examples.
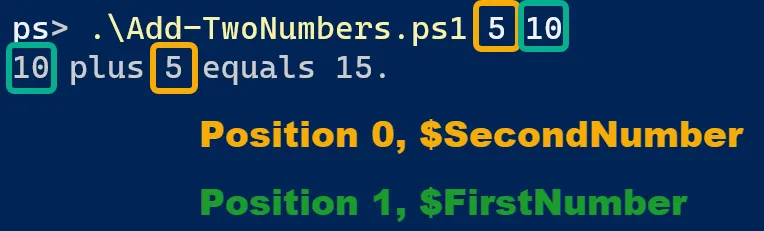
Parameter Aliases
Parameters can also have aliases or other names. When executing the cmdlet or script, you use the alias instead of the parameter name. Aliases are great for shortening parameter names, having multiple parameter name options, or allowing the parameter to accept different object types from the pipeline.
You define an alias by declaring the Alias attribute above the parameter declaration. Aliases are enclosed in quotes and separated by commas. The updated script parameter definition is below, along with screenshots demonstrating their usage.
param(
[Alias("Num1","FirstNum")]
[Parameter(
Mandatory,
Position = 0
)]
[double]$FirstNumber,
[Alias("Num2","SecondNum")]
[Parameter(
Mandatory,
Position = 1
)]
[double]$SecondNumber
)

Parameter Default Values
Parameters can also have default values. Default values are helpful because they reduce the required inputs and provide default behavior for the script or cmdlet. Default values also ensure that any critical parameters always have a value.
You assign a default value to a parameter using the equal sign (=
) next to the parameter name, just like you would when assigning a value to a variable. In the example code below, $SecondNumber
default value is 0
. Running the script with this parameter allows the script to run still and output the result.
Note: When assigning a default value to a parameter, the parameter should not be mandatory. If it is, PowerShell still prompts for the parameter value when executing the cmdlet or script.
param(
[Alias("Num1","FirstNum")]
[Parameter(
Mandatory,
Position = 0
)]
[double]$FirstNumber,
[Alias("Num2","SecondNum")]
[Parameter(
Position = 1
)]
[double]$SecondNumber = 0
)

Summary
In conclusion, mastering PowerShell script parameters is fundamental for effective PowerShell scripting. Parameters facilitate user interaction, enhance script flexibility, and ensure script reliability. PowerShell script authors can create more robust and versatile scripts that meet various needs and scenarios by learning to define, customize, and utilize parameters effectively.
Have you enjoyed this article? Read more of my PowerShell content here!