Creating an SSH key is an excellent authentication method when working with GitHub. However, sometimes you need to work with multiple GitHub accounts on the same system, like a work account and a personal account. To accomplish this, you can create multiple SSH keys and associate each one with different GitHub accounts.
In this tutorial, you will learn how to configure and manage these multiple accounts and SSH keys for authenticating with multiple GitHub accounts. To follow along, you will need:
- Multiple GitHub accounts (sign up here)
- Download and install the Git utility
- Existing SSH public key associated with one GitHub account
Need to learn the basics of SSH authentication with GitHub? Check out this tutorial titled How to Implement GitHub SSH Authentication!
Generate Second SSH Key
This tutorial assumes you already use an SSH key with an existing GitHub account. To use a second GitHub account for authentication, generate a second SSH key with a different file name than the first key.
For example, your first SSH key might have files names such as id_rsa
(private key) and id_rsa.pub
(public key). To generate a second set of SSH keys, use the ssh-keygen
command and specify:
- Key type (
-t rsa
) - File name in the .ssh folder in your home directory (
-f ".ssh/id_rsa_jeff2"
) - Comment with your email address (
-C "jeff2@jeffbrown.tech"
)
When prompted to enter a passphrase, enter a passphrase, or press Enter to leave it blank. Adding a passphrase adds security to the SSH key but is not required. This tutorial is leaving the passphrase blank.
ssh-keygen -t rsa -f ".ssh/id_rsa_jeff2" -C "jeff2@jeffbrown.tech"
At this point, you should have two sets of SSH keys saved in the .ssh
folder in your home directory, both a private and public key pair.
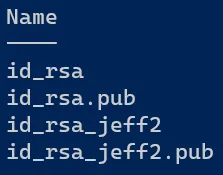
Register New Key with SSH Agent
Next, add the SSH key to the SSH agent. The SSH agent manages your SSH key and remembers your passphrase, so you don’t have to reenter it each you use your SSH key. First, ensure the SSH agent is running using the eval command, then use the ssh-add
command and specify the file name created in the previous step (~/.ssh/id_rsa_jeff2
).
eval $(ssh-agent)
ssh-add ~/.ssh/id_rsa_jeff2
Add SSH Key to Second GitHub Account
Add the public key to your other GitHub account. For more information on how to do this, check out these links:
Jeff Brown Tech | Add SSH Key to GitHub Account
GitHub Docs | Adding a new SSH key to your GitHub account
Create an SSH Config File
With both sets of SSH keys created and added to different GitHub accounts, how does Git know when the correct credentials? The answer is an SSH config file. The SSH config file sets configuration rules that specify when to use each account. You set the credentials based on the domain name.
In your home directory in the .ssh
folder, create a new file named config
(no file extension). In BASH, you can use the touch
command, and in PowerShell, you can use the New-Item
command to create new files.
# BASH example
touch ~/.ssh/config
# PowerShell example
New-Item .\.ssh\config -ItemType File
Open the file using any text editor or IDE. On Linux, this could be something like vim or nano. On Windows, you can use Notepad or something more advanced like VS Code.
Create profiles that specify when to use each identity or SSH key in the config file. The profiles should include:
- Host: The host name from the Git URL used to clone the repo.
- HostName: The real host name used to log in to. Since this tutorial uses GitHub, the host name here will be
GitHub.com
. - User: User ID, which will be
git
. - IdentityFile: Path to the SSH public key file to use for authentication.
Here is an example config file with two profiles:
- The default configuration for work, which uses the private key file
~/.ssh/id_rsa
. This profile should be the one you use the most often. - A second personal profile, which uses the private key file
~/.ssh/id_rsa_jeff2
.
Note the different Host
values of github.com
and github.com-jeff2
. These hostnames are how Git identifies which SSH key to use when cloning the repository.
# Default Config - Work profile
Host github.com
HostName github.com
User git
IdentityFile ~/.ssh/id_rsa
# Secondary Config - Personal profile
Host github.com-jeff2
HostName github.com
User git
IdentityFile ~/.ssh/id_rsa_jeff2
Cloning Repositories
The real fun beings when cloning repositories. Using the example config file above, you use the same repository URL if you are cloning a repository from your work GitHub. This example URL is for a repository named 100DaysOfCloud from the account named JeffBrownTech:
git@github.com:JeffBrownTech/100DaysOfCloud.git
However, to clone a repository using the personal profile from the config file, you need to change the hostname in the clone URL to match the hostname in the config file. Here is another example of a clone URL; note the modified hostname github.com-jeff2. This hostname matches the Host value defined in the config file.
git@github.com-jeff2:JeffBrown2/myCode.git
By changing the hostname in the repository’s clone URL, Git knows to use the secondary profile from the config file, which uses a different SSH private key.
After you clone each repository, you can change the username and email address Git uses when creating commits. You may have already configured a global username and email address, but Git allows setting these values per repository. Use the git config
commands to set these values.
git config user.name "<Your Name>"
git config user.email "<Your Email Address>"
Summary of SSH with Multiple GitHub Accounts
In this tutorial, you learned how to generate a second set of SSH keys to authenticate to GitHub using different accounts. Authenticating with multiple SSH keys for different accounts is made possible through the config file by defining different hostnames.
Questions or feedback? Leave a note below or say “hi” on Twitter.
References:
SSH config file syntax and how-tos for configuring the OpenSSH client
How to Configure Git Username and Email Address | Linuxize
Adding a new SSH key to your GitHub account – GitHub Docs