PowerShell arrays are a powerful construct when working with a group of similar items. You can loop through them and access items using their index values. However, adding or removing items to a fixed array is not intuitive and, depending on the method, can cause performance issues. This article will teach you different strategies for managing dynamic arrays in PowerShell.
What is an array?
Arrays are a common data type found in many programming languages. An array is a collection of values or objects you can sort, add, or remove items. The array items can be of the same type, like numbers or strings, or different types.
There are several ways to create an array. You can input a list of items separated by commas or enclose the items in an ampersand and parentheses @()
. Both PowerShell commands below create the same array names $animals
.
$animals = 'cat','dog','bird','horse','rabbit'
$animals = @('cat','dog','bird','horse','rabbit')
You can use various methods to access the array elements. You can use index values starting at 0 or even a range of values. For example, the first command retrieves the array item at index 1, which is dog. The second command retrieves items at index 2 through 4: bird, horse, rabbit. A neat trick is to use -1 to access the last element in the array if you do not know how many items are in the array.
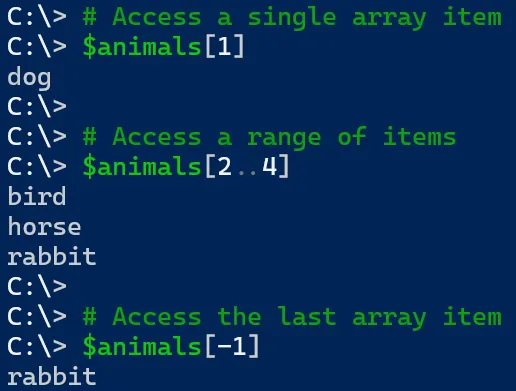
Managing Fixed Sized Arrays
With a basic definition of arrays out of the way, let’s discuss how to manage array items. By default, arrays are of fixed size, meaning you cannot easily add or remove items. You can tell if an array is of fixed size by viewing IsFixedSize
on the array. If you try to use the Add()
method on an array, PowerShell displays an error message saying the collection was of a fixed size.

You can use the +=
operator to add items to the array. PowerShell adds an item by copying the array with the original values, then adding the new value. However, if you use this method several times in a script or the array is very large, this method can cause performance issues and should be avoided if possible. The screenshot below initializes the $animals
array, then adds fox to the array using the +=
method.
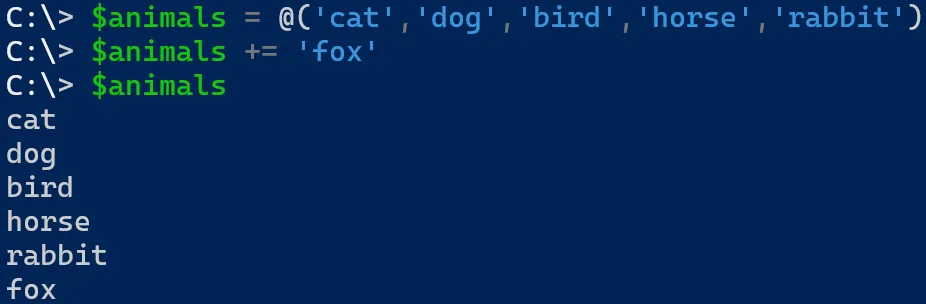
You cannot use the -=
operator to remove array items. However, you can remove values by using the -ne
(not equal) operator. For example, to remove bird from the $animals
array, set $animals
equal to the array where values are not equal to bird
.
$animals = $animals -ne 'bird'
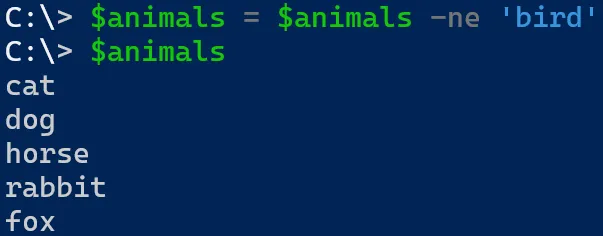
Managing PowerShell Dynamic Arrays
In the previous section, you learned about fixed sized arrays. While there are methods to add and remove array items, neither is very intuitive. Instead of dealing with fixed sized arrays, you can use .NET classes in PowerShell to manage dynamic arrays.
ArrayList
The ArrayList object enables quickly adding and removing items in a collection. This ability overcomes the limitations of adding and removing items from earlier in the article. You use the Add()
and Remove()
methods when managing items in the array.
To define an ArrayList, use the Systems.Collection
namespace with ArrayList
. Let’s declare a blank animal collection using [System.Collection.ArrayList]
along with the default constructor. Next, use Add()
to add items to the collection. Finally, output the collection to the console to view the items.
$animalsArrayList = [System.Collections.ArrayList]::new()
$animalsArrayList.Add('cat')
$animalsArrayList.Add('dog')
$animalsArrayList.Add('bird')
$animalsArrayList
PowerShell outputs the item’s index to the console when adding items to the collection. Review the screenshot below where adding ‘cat’ outputs 0, ‘dog’ is 1, and ‘bird’ is 2.
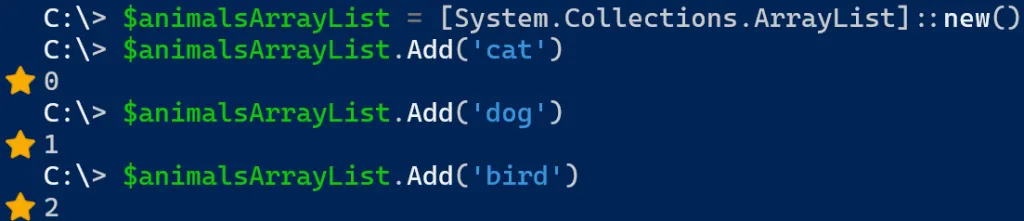
If your script is running non-interactively, this output will not affect anything. However, this output can clutter the console if you watch the script in real-time. One method to suppress this output is to add [void]
when invoking the add method.
$animalsArrayList = [System.Collections.ArrayList]::new()
[void]$animalsArrayList.Add('cat')
[void]$animalsArrayList.Add('dog')
[void]$animalsArrayList.Add('bird')
You can also invoke the Remove()
method to remove items from the collection. Reference the item by its name, and there is no need to suppress any output from the command. The following example removes ‘dog’ from the collection.
$animalsArrayList.Remove('dog')
If you have an existing fixed sized array, you can convert it to an ArrayList using the following methods. First, you can define a new ArrayList based on the contents of the original array. The following example illustrates a fixed array named $fixedArray
with our animal items. The second line creates $arrayList
of [System.Collections.ArrayList]
type and sets its value to the original $fixedArray
.
$fixedArray = @('cat','dog','bird','horse','rabbit')
[System.Collections.ArrayList]$arrayList = $fixedArray
Generic List
Another option is to use a generic list. Generic lists require defining the data type used in the collection, such as int
or string
. Here are some examples that illustrate an empty generic list of these types.
# Define generic string list
$myStringList = [System.Collections.Generic.List[string]]::new()
# Define generic int list
$myIntList = [System.Collections.Generic.List[int]]::new()
You can use the Add()
and Remove()
methods to manage the list items. However, unlike with ArrayList, you do not need to void the output when adding items. However, removing items does output True
or False
if it found a matching item, so void the output on those actions to suppress console output.
$myStringList.Add('string1')
$myStringList.Add('string2')
[void]$myStringList.Remove('string2')
$myIntList.Add(1)
$myIntList.Add(3)
[void]$myIntList.Remove(1)
Like the ArrayList example earlier, you can convert an existing fixed array into a generic list by creating a new list based on the array’s values. Remember, for generic lists, you need to define the data type stored in the list.
$fixedArray = @('cat','dog','bird','horse','rabbit')
[System.Collections.Generic.List[string]]$genericList = $fixedArray
Summary
Using PowerShell fixed arrays can meet most of your needs. However, if you need to add or remove elements, it is better to use ArrayList or Generic list in PowerShell to manage the items dynamically.
Enjoyed this article? Check out more PowerShell content here!