Managing secrets, passwords, or tokens in a PowerShell script has always been a tricky situation. Microsoft has released the PowerShell SecretManagement module. This module provides cmdlets to interact with secrets across multiple types of vaults, so you can write a PowerShell script once that works with many solutions.
In this article, you will learn about the SecretManagement module and the SecretStore module, a Microsoft-produced extension vault. You can then follow along with a tutorial on installing and registering a local secret vault on your system.
Check out more great PowerShell content here!
Introducing SecretManagement PowerShell Module
The SecretManagement module allows PowerShell to store and retrieve secrets from a local or remote vault. You register vaults containing secrets utilized by your PowerShell script or module. The SecretManagement module provides a layer of abstraction between PowerShell and the vault storing the secret.
You can install SecretManagement from the PowerShell Gallery using the following command:
Install-Module Microsoft.PowerShell.SecretManagement
The SecretManagement module provides all the cmdlets to register, store, and retrieve secrets from a vault. This single set of PowerShell cmdlets works across multiple vault types.
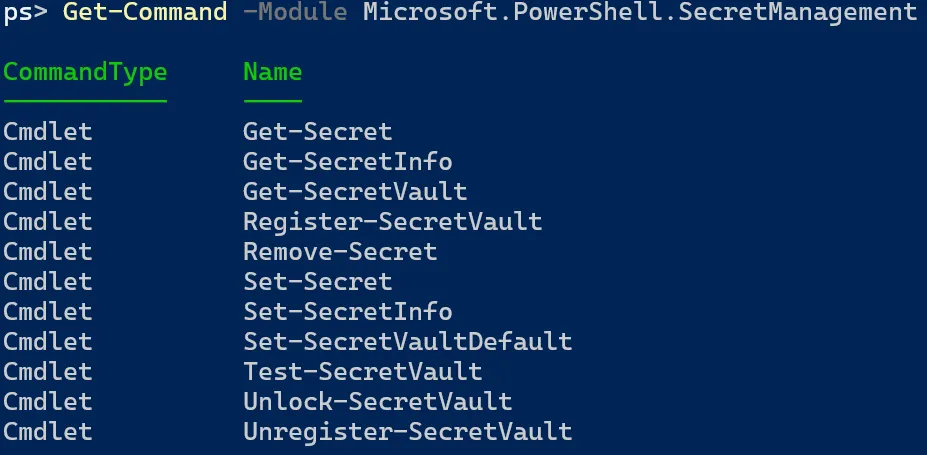
Exploring the Extension Vault Ecosystem
Extension vaults are PowerShell modules that connect the SecretManagement module and a local or remote vault. To find PowerShell modules that support SecretManagement, search the PowerShell Gallery for the “SecretManagement” tag or visit https://aka.ms/SecretManagementVaults. Vault extensions written by the community include:
- Azure Key Vault (Microsoft owned)
- BitWarden
- CyberArk
- HashiCorp Vault
- KeePass
- Keeper
- LastPass
- macOS KeyChain
- SecretStore
The SecretManagement module does not dictate a specific authentication method for extension vaults. Each vault provider provides its own authentication mechanism with some requiring a password, token, or other account credentials. Changes to the vault’s authentication method will not impact the script as the module interacts with the vault on behalf of the script to retrieve the secrets.
This extensible model gives you more flexibility as a PowerShell coder when writing or sharing a script. You can write a script that retrieves a secret from a vault, then share this script with others without worrying about where the secret is stored.
You can also use the same script across multiple environments, such as your local system, test, or production. You only need to change the registered vault name used in the -Vault
parameter.
Introducing SecretStore Extension Vault
Along with the SecretManagement module, Microsoft introduced the SecretStore extension vault. SecretStore allows you to register, store, and retrieve secrets on the local machine using the currently logged-on user account. SecretStore encrypts secrets on file using .NET Crypto APIs, and secrets stay encrypted while in memory. This module works on all supported PowerShell Windows, Linux, and macOS platforms.
You can install the SecretStore module from the PowerShell Gallery using the following command:
Install-Module Microsoft.PowerShell.SecretStore
The module provides the following cmdlets for managing SecretStore:
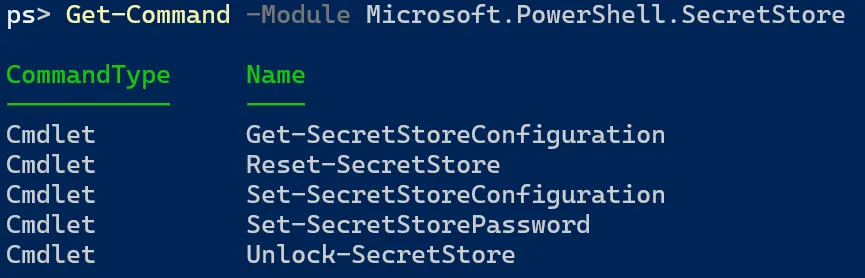
Manage SecretStore Interactively
With both modules, you can create a vault on your local system using SecretStore, then register the vault using SecretManagement. You can then create and retrieve encrypted secrets on your local system.
To get started, first review the default settings for the SecretStore by running Get-SecretStoreConfiguration
. If this is the first time running the command, PowerShell prompts you to set a password. This password is used to unlock the vault.
In the screenshot below, the SecretStore was already configured, so PowerShell prompted for the password. Some of the SecretStore settings include:
- Scope: the context the SecretStore operates. Only CurrentUser is currently supported and cannot be changed.
- Authentication: how to authenticate to access the SecretStore. Valid options are Password (default) or None.
- PasswordTimeout: how many seconds the vault remains unlocked after successful password authentication. When this timeout has expired, the current session becomes invalid and requires the password again. The default is 900 seconds.
- Interaction: specifies whether the SecretStore prompts the user when a secret is being set or retrieved. The value Prompt means the user is prompted for the password in an interactive session. The value None means the user is not prompted for a password. If you set the value to None, then also set the Authentication value to None, or PowerShell will throw a Microsoft.PowerShell.SecretStore.PasswordRequiredException error.
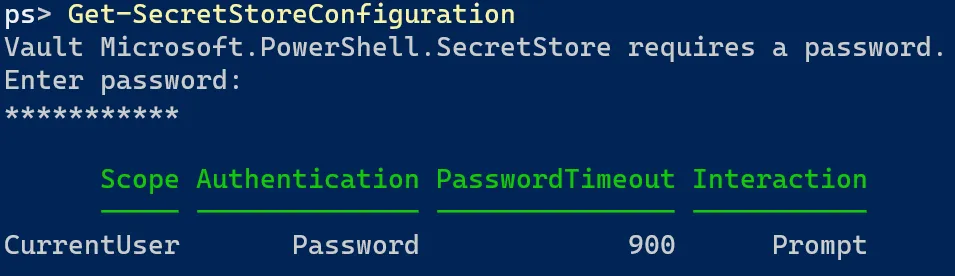
Modify PasswordTimeout
For this tutorial, use the Set-SecretStoreConfiguration
cmdlet to set the PasswordTimeout
to 30
seconds. This change ensures frequent password prompt so you can see the authentication prompts when interacting with the vault.
Set-SecretStoreConfiguration -PasswordTimeout 30
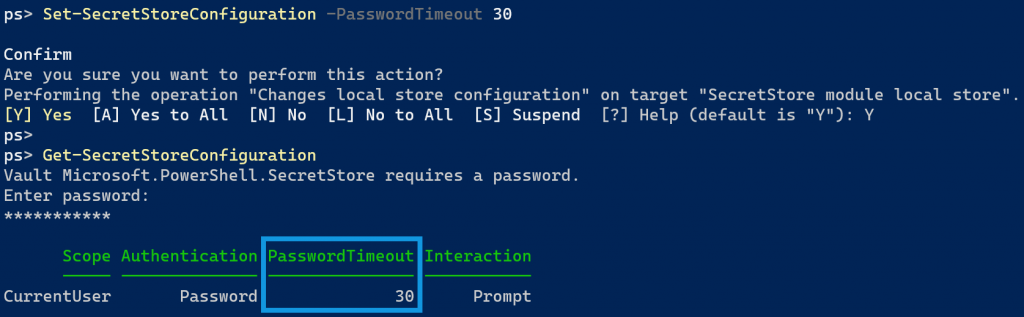
Register SecretStore vault
The next step is to register the SecretStore vault with the SecretManagement module. Registering allows the SecretManagement module to set and retrieve secrets from the local SecretStore vault.
Use the Register-SecretVault
command and specify the Name
of the vault (MyLocalSecretStore
), the ModuleName
providing access to the vault (Microsoft.PowerShell.SecretStore
), and use DefaultVault
to set the vault as default for the current user.
Register-SecretVault `
-Name MyLocalSecretStore `
-ModuleName Microsoft.PowerShell.SecretStore `
-DefaultVault
Set and Retrieve Secrets
With the local SecretStore vault configured and registered with the SecretManagement module, you can now set and retrieve secrets. Use cmdlets from the SecretManagement module to set and retrieve secrets stored in your local SecretStore vault.
First, use the Set-Secret
cmdlet and specify the secret Name
(MySecret1
) and the Secret
value (SuperDuperPassword!
). The Secret parameter accepts the following data types:
- Byte[]
- String
- SecureString
- PSCredential
- Hashtable
Note: If the vault is locked, PowerShell prompts you for the vault password configured when you ran Get-SecretStoreConfiguration. If this initial setup did not occur, the SecretVault will now ask you to set a password for the vault.
Set-Secret -Name "MySecret1" -Secret "SuperDuperPassword!"
Next, use the Get-Secret
cmdlet to retrieve the secret value by specifying the Name
of the secret (MySecret1
). By default, SecretManagement returns the secret as a SecureString. If you need the secret value in plain text, use the AsPlainText
parameter.
# Retrieve secret as SecureString
Get-Secret -Name "MySecret1"
# Retrieve secret in plain text
Get-Secret -Name "MySecret1" -AsPlainText
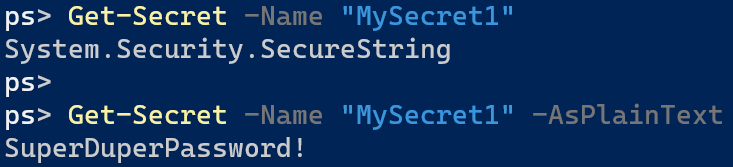
Metadata
You can associate metadata with the secret. However, not all vaults support metadata, so review your preferred vault’s documentation. Metadata values must be a string, int, or DateTime type.
To add metadata to a secret, create a hashtable containing key-value pairs, for example, when the secret was last changed. Create a hashtable named $metadata
with a key of LastChanged
and a value using the command Get-Date
in the Format yyyy-MM-dd.
$metadata = @{ LastModified = (Get-Date -Format yyyy-MM-dd) }
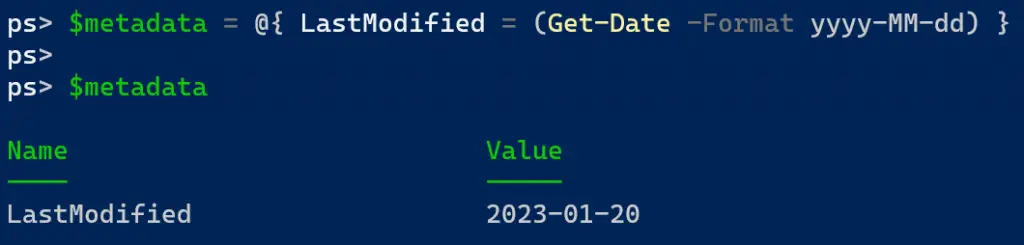
Learn more about hashtables in PowerShell Hash Table vs. PSCustomObject: Deep Dive & Comparison
Next, use the Set-Secret
command again, this time specifying Metadata using the hashtable you just created ($metadata
).
Set-Secret `
-Name "MySecret1" `
-Secret "SuperDuperPassword!" `
-Metadata $metadata
You can then view the metadata using the Get-SecretInfo
command specifying the secret Name
(MySecret1
) and Format-List
to view the metadata.
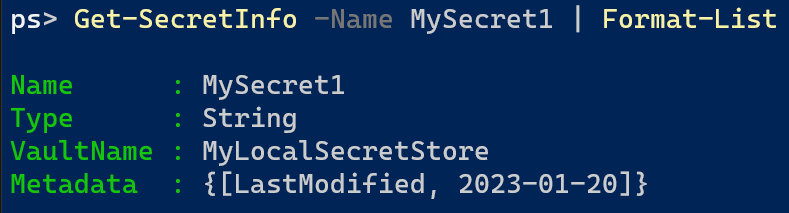
Unlock vault
If the vault is locked when you try to create, set, or get a secret, SecretStore prompts for the vault password. However, you can proactively unlock the vault using the Unlock-SecretStore
command.
You can enter the password when prompted or use the Password
parameter. The screenshot below shows SecretManagement prompting for the password, then retrieving a secret.
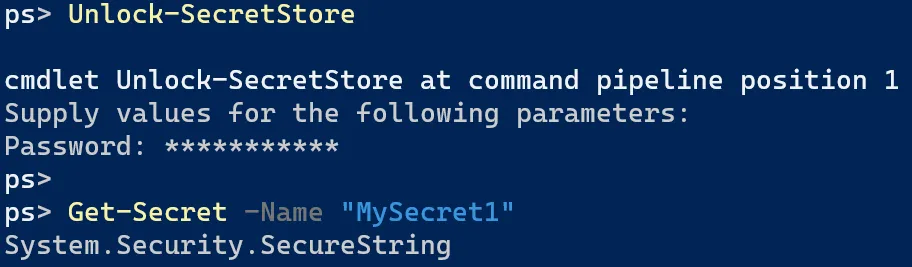
The vault will relock after the vault’s configured timeout. However, you can unlock the vault manually and specify a new PasswordTimeout value in seconds. The PasswordTimeout parameter overrides the vault’s configured password timeout value. The screenshot below shows unlocking the vault for 300 seconds or 5 minutes.

SecretManagement PowerShell Module Summary
The SecretManagement module lets you use PowerShell to interact with other vaults to get, set, and retrieve secrets. The module provides an abstraction layer, so you don’t have to know the details of what the vault is or how it authenticates. Extensions vault modules provide this information and are available for several popular solutions. Microsoft released the SecretStore extension vault module as a way to encrypt secrets on your local file system.