Are you tired of applying a Terraform configuration only to find one of your variable values doesn’t work? Sounds like you need Terraform variables validation!
In this post, you will learn all about the validation syntax and how to use validation in a few different scenarios for an Azure storage account deployment.
Want to learn about environment variables? Check out my other article Getting to Know Terraform Environment Variables!
Working with Terraform Variables Validation
When declaring Terraform variables, you have a few options for defining what the variable looks like. You set the variable type (string, number, bool, etc.), a description of the variable, a default value to make the variable optional, and whether or not the variable contains a sensitive value, like a password.
Terraform variables also have a validation
argument. The validation
argument defines rules to determine if the variable value meets certain requirements. These requirements could be a specific length for a string or ensuring the value starts with a specific prefix.
The validation rules use the built-in Terraform functions to validate the value. For example, use the length()
function to validate if a string contains a specific number of characters. If you want to test your validation rules, use the terraform console
to open the Terraform expression console in your favorite shell environment.
Validation rules have two parts:
- Condition: The condition uses Terraform functions to perform validation on the variable value. You can put multiple statements together using two ampersands (&&) for an
and
statement or two pipe symboles (| |) for anor
statement. Inside the condition, you can only reference the current variable, not other variables in the configuration. - Error message: Terraform displays the error message when the validation fails. The validation error message must be at least one full sentence starting with an uppercase letter and ending with a period or question mark.
Here is the basic syntax for declaring a variable and including the validation argument with the two parts. In this example, the validation’s condition checks to make sure resource_name
is greater than 5 characters in length. When referencing the variable in the condition
argument, prepend the variable name with var.
, just as you would in a resource definition. The error_message
argument is a complete sentence outlining why the validation failed.
variable "resource_name" {
type = string
description = "Input name of the resource."
validation {
condition = length(var.resource_name) > 5
error_message = "The resource_name must be greater than 5 characters in length."
}
}
Terraform Variable Validation Examples
Here are a few examples of how to validate your Terraform variables using a storage account resource as an example.
Validating Azure Storage Account Names
Azure storage account names must be globally unique and between 3 to 24 characters. Using the length()
function, configure a validation rule that ensures the variable value is between these lengths. Use double ampersands (&&) to put multiple rules together. Add a custom error message as well.
variable "storage_account_name" {
type = string
description = "Globally unique name for the storage account."
validation {
condition = length(var.storage_account_name) >=3 && length(var.storage_account_name) <= 24
error_message = "The storage_account_name variable name must be 3-24 characters in length."
}
}
Perhaps you also want the storage account to have a certain prefix, like a company identifier. Add a second validation block in the variable definition to verify the prefix exists. In this example, use the regex()
function to test the pattern (^jbt
) is at the beginning of the string value stored in var.storage_account_name
.
variable "storage_account_name" {
type = string
description = "Input name of the resource."
validation {
condition = length(var.storage_account_name) >= 3 && length(var.storage_account_name) <= 24
error_message = "The storage_account_name variable name must be 3-24 characters in length."
}
validation {
condition = can(regex("^jbt", var.storage_account_name))
error_message = "The storage_account_name variable must have a 'jbt' prefix."
}
}
Here are the validation rules in action, both checking for the proper prefix and name length.
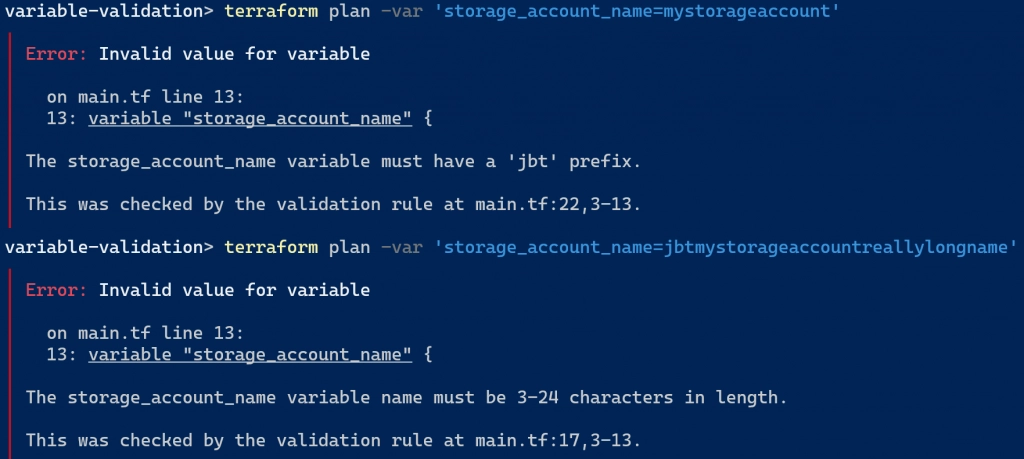
Validating Azure Storage Account SKU
Terraform does not provide a way to set an allowed values list for a variable value. However, you can use a validation condition to ensure the variable value only contains certain options.
You can provision storage accounts in one of two SKUs: hot or cool. Using a validation rule, you make sure that incoming variable values only match one of these options. Use double pipe symbols (| |) as an OR statement to say the value can match any of these strings.
variable "storage_access_tier" {
type = string
description = "Access tier for storage account. Must be Hot or Cool."
validation {
condition = var.storage_access_tier == "Hot" || var.storage_access_tier == "Cool"
error_message = "The storage account must be set to Hot or Cool."
}
}
Here is the validation in action when trying to set the access tier to “Archive”.
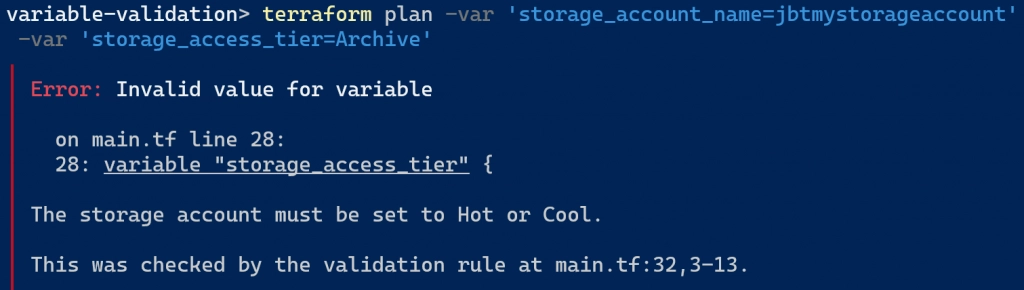
The downside of this method is the variable value must be case-sensitive. If the Terraform configuration tried to use “hot” or “cool”, the validation fails.
To see the full examples from above, check out this GitHub repository:
GitHub | JeffBrownTech | terraform_learning | variable-validation | main.tf
Closing
Variable validation is a great tool to ensure the variable input Terraform receives doesn’t result in a deployment error. The built-in Terraform functions can be limiting but provide opportunities to find unique solutions to perform the validation.