PowerShell 7 introduced the ternary operator. The ternary operator is available in other programming languages, notably C#. The ternary operator can be confusing if you are unfamiliar with its syntax.
In this tutorial, you will learn all about the ternary operator, how to create one in PowerShell 7, and some use cases to get you started. For this tutorial, you will need any PowerShell version 7 installed on your system.
What is a ternary operator?
Let’s look at an if-else
statement that tests if the number stored in $valueA
is greater than another number stored in $valueB
.
$valueA = 50
$valueB = 100
if ($valueA -gt $valueB) {
Write-Host "$valueA is greater than $valueB."
}
else {
Write-Host "$valueA is less than $valueB."
}
In PowerShell 7, you can use a ternary operator. A ternary operator is a simplified if-else
statement. PowerShell’s ternary operator is modeled and uses similar syntax you see in C#.
Here is an outline of the syntax. You still have the tested condition and two actions. The question mark (?
) indicates the then
statement, and the colon (:
) means the else
statement.
If the <condition>
is $true
, then PowerShell runs the statement after the question mark.
If the <condition>
is $false
, then PowerShell runs the statement after the colon.
If the <condition>
evaluates to $null
, then PowerShell runs the <if-false statement>
section.
<condition> ? <if-true statement> : <if-false statement>
Here’s what the original if-else
statement looks like written in ternary syntax. The first example includes the Write-Host
command, while the second does not. Note that parentheses ( )
are not required if the then
or else
statement is simple.
$valueA = 50
$valueB = 100
# Using complex then/else statements
$valueA -gt $valueB ? (Write-Host "$valueA is greater than $valueB.") : (Write-Host "$valueA less than $valueB.")
# Using simple then/else statements
$valueA -gt $valueB ? "$valueA is greater than $valueB." : "$valueA less than $valueB."
The ternary operator allows removing if
and else
keywords along with braces { }
denoting code blocks. Ternary operators are meant for simpler if-else
statements that do not use multiple lines of code for the then
or else
statements.
PowerShell 7 ternary operator use cases
The following sections outline a few use cases of the ternary operator.
Stopping services
Ternary operators are great for testing service status. For example, you test if a service is currently running. If it is, stop the service. If it is not, display a message or start the service.
Here the condition is testing for the Print Spooler (or Spooler
) service and if it is currently in Running status. If the service is running, use the Stop-Service
command to stop the service. If the service is not running, display a message of Service not started
.
$service = Get-Service -Name 'Spooler'
$service.Status -eq 'Running' ? (Stop-Service -Name 'Spooler') : 'Service not started'
Testing file paths
A common PowerShell task is testing whether a folder or file exists and taking some action. This example tests if a directory exists (C:\logFiles
) using the Test-Path
command. If it does exist, display a message that the directory already exists. If it does not exist, use the New-Item
command to create the directory.
(Test-Path -Path 'C:\logFiles') ? 'Directory already exists' : (New-Item -Path 'C:\logFiles' -ItemType Directory)
Testing operating system type
PowerShell 7 includes three automatic variables for testing operating system type: $IsLinux
, $IsMacOS
, and $IsWindows
. Use these in a simple ternary statement to test for the client operating system.
$IsWindows ? 'You are running Windows' : 'You are not running Windows'
$IsMacOS ? 'You are running MacOS' : 'You are not running MacOS'
$IsLinux ? 'You are running Linux' : 'You are not running Linux'
Custom object values
Ternary operators are great for calculating values in a PowerShell custom object. This example contains an array of objects, and each object has three properties: Name
, Price
, and OnSale
.
$widgetArray = @(
[PSCustomObject]@{
Name = "widget001"
Price = 5.00
OnSale = $true
},
[PSCustomObject]@{
Name = "widget002"
Price = 13.00
OnSale = $true
},
[PSCustomObject]@{
Name = "widget003"
Price = 20.00
OnSale = $false
}
)
Learn the difference between PowerShell hash tables and PSCustomObjects in PowerShell Hash Tables vs. PSCustomObject: Deep Dive & Comparison.
In a foreach
loop, create a new PowerShell custom object with calculated values based on the array. For example, if the price is over $10 or the item is currently on sale. If the item is on sale, calculate the new sale price. The ternary operator simplifies the calculation and easily fits next to the object’s key name. The image after the code snippet shows the output of the foreach
loop.
foreach ($widget in $widgetArray) {
[PSCustomObject]@{
"Name" = $widget.Name
"Over10Dollars" = ($widget.Price -gt 10) ? $true : $false
"OnSale" = ($widget.OnSale) ? 'On Sale' : 'Not on Sale'
"SalePrice" = ($widget.OnSale) ? $widget.Price * 0.85 : "---"
}
}
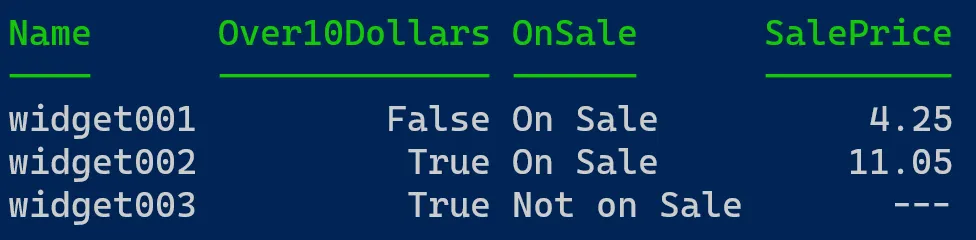
PowerShell 7 ternary notes
Multiple lines
PowerShell 7 interprets the question mark and the colon in the ternary operator as a line continuation. For example, take the testing file paths example from above. You can split the condition and each statement on separate lines, like this:
# Using ternary syntax for line continuation
(Test-Path -Path 'C:\logFiles') ?
'Directory already exists' :
(New-Item -Path 'C:\logFiles' -ItemType Directory)
Nesting ternary operators
You can nest a ternary operator within a ternary operator. Take the operating system examples from earlier. Instead of three separate statements, you can start another ternary operator in the <if-false>
statement block.
$IsMacOS ? 'You are running MacOS' : $IsLinux ? 'You are running Linux' : 'You are running Windows.'
If using tradition if/else
syntax, this statement looks like this:
if ($IsMacOS) {
'You are running MacOS'
}
elseif ($IsLinux) {
'You are running Linux'
}
else {
'You are running Windows.'
}
PowerShell 7 Ternary Operator FAQs
What is a ternary operator?
A ternary operator uses three arguments (or operands) to evaluate a Boolean expression and returns one of the values.
What is the PowerShell 7 ternary operator syntax?
The PowerShell 7 ternary operator syntax is <condition> ? <if-true statement> : <if-false statement>
.
Conclusion
The PowerShell 7 ternary operator uses simpler syntax to create an if-else
statement. While the syntax is more straightforward, people unfamiliar with the ternary syntax may find it confusing. The shorter syntax has its use cases. Unfortunately, you cannot use it in earlier PowerShell versions, making compatibility difficult if you write scripts for systems using older versions.
Enjoyed this article? Check out more of my PowerShell content here!